In this guide, you will learn how to authenticate users in an Astro application using Clerk and React. You will go through the process of setting up a new Astro project, enabling server-side rendering using Node.js adapter, and integrating Clerk to authenticate users seamlessly.
Prerequisites
You’ll need the following:
- Node.js 18 or later
- A Clerk account
Table Of Contents
- Create a new Astro application
- Integrate React in your Astro project
- Integrate Node.js adapter in your Astro project
- Create a new Clerk application
- Integrate Clerk in your Astro project
- Build and Test your Astro application locally
Create a new Astro application
Let’s get started by creating a new Astro project. Execute the following command:
npm create astro@latest my-app
npm create astro
is the recommended way to scaffold an Astro project quickly.
When prompted, choose:
Empty
when prompted on how to start the new project.Yes
when prompted if plan to write Typescript.Strict
when prompted how strict Typescript should be.Yes
when prompted to install dependencies.Yes
when prompted to initialize a git repository.
Once that’s done, you can move into the project directory and start the app:
cd my-app
npm run dev
The app should be running on localhost:4321.
Now, let’s move on to integrate React in your Astro application.
Integrate React in your Astro project
To create dynamic user interfaces with Clerk, you will integrate React in your Astro project. Execute the following command:
npx astro add react
npx
allows us to execute npm packages binaries without having to first install it globally.
When prompted, choose the following:
Yes
when prompted whether to install the React dependencies.Yes
when prompted whether to make changes to Astro configuration file.Yes
when prompted whether to make changes totsconfig.json
file.
Now, let’s move on to enabling server-side rendering in your Astro application.
Integrate Node.js adapter in your Astro project
To authenticate and maintain user sessions with Clerk, you will enable server-side rendering in your Astro project via the Node.js adapter. Execute the following command:
npx astro add node
When prompted, choose the following:
Yes
when prompted whether to install the Node.js dependencies.Yes
when prompted whether to make changes to Astro configuration file.
Now, let’s move on to creating a new Clerk application.
Create a new Clerk application
- In your Clerk Dashboard, to create a new app, press the + New application card to interactively start curating your own authentication setup form.
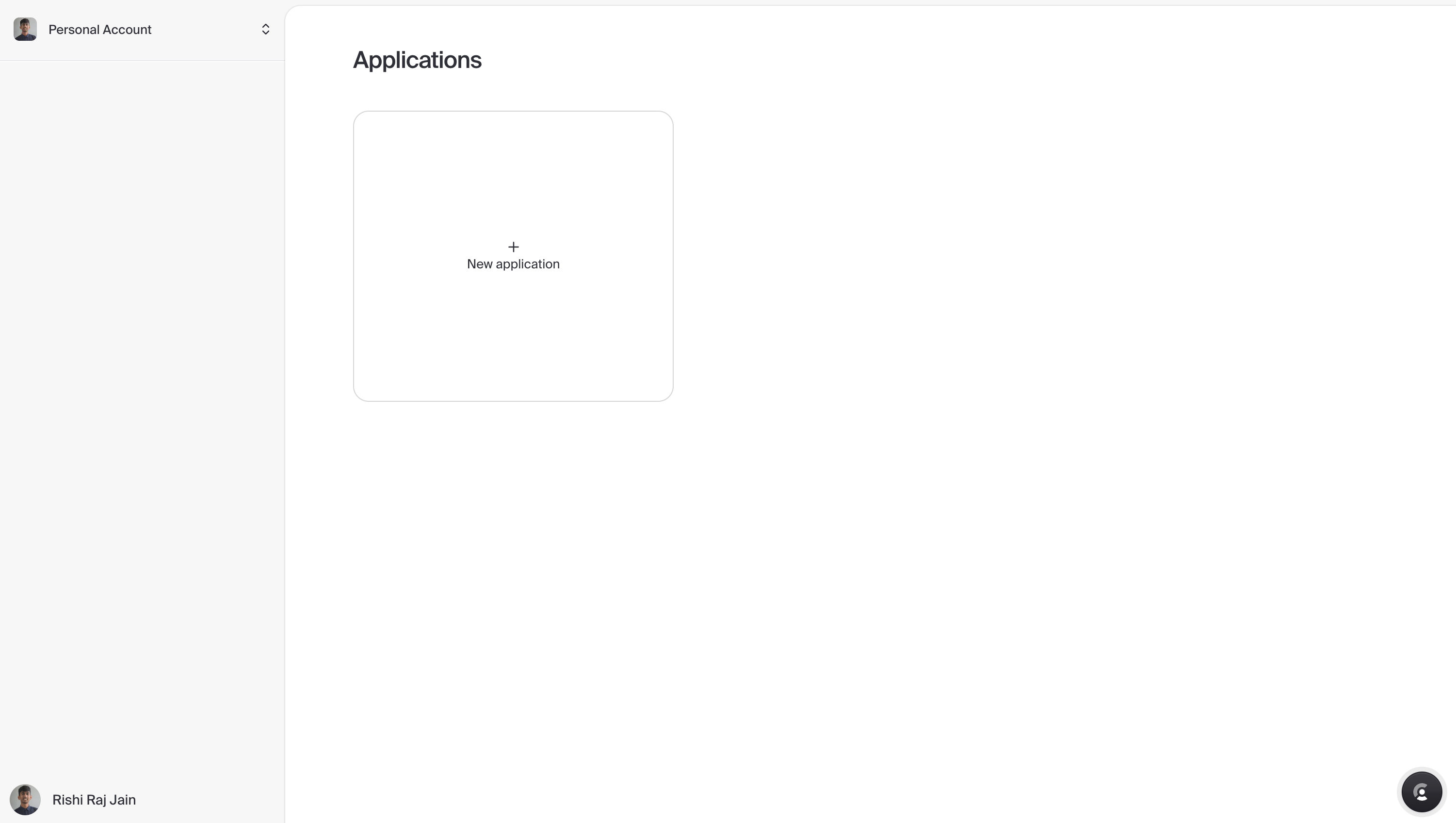
- With an application name of your choice, enable user authentication via credentials by toggling on Email and allow user authentication via Social Sign-On by toggling on providers such as Google, GitHub and Microsoft.
.png)
- Once the application is created in the Clerk dashboard, by default you will be shown with your application’s API keys for Next.js. Copy and securely store these API Keys in a file named
.env
to be used in your Astro application as the following:
# .env
# Clerk Environment Variables
PUBLIC_ASTRO_APP_CLERK_PUBLISHABLE_KEY="pk_test_..."
CLERK_SECRET_KEY="sk_test_..."
.png)
Now, let’s move on to integrate Clerk in your Astro project.
Integrate Clerk in your Astro project
Install the Clerk’s Community SDK for Astro
Execute the command below to install the necessary package for building authentication with Clerk:
npm install astro-clerk-auth
The command installs the following library:
astro-clerk-auth
: A package that integrates Clerk with Astro via Middleware support and baked-in React and Astro components for Clerk.
Further, perform the following additions in astro.config.mjs
to use the Clerk integration:
// File: astro.config.mjs
import node from "@astrojs/node";
import react from "@astrojs/react";
import clerk from "astro-clerk-auth";
import { defineConfig } from "astro/config";
export default defineConfig({
output: "server",
adapter: node({
mode: "standalone",
}),
integrations: [
react(),
clerk({
afterSignInUrl: "/",
afterSignUpUrl: "/",
}),
],
});
Intercept all incoming requests using Astro middleware
To make sure that each request maintains a user session accessible over the server-side endpoints, and in .astro
pages during server-side rendering, you are going create a middleware that uses Clerk to decode/encode a user session from the cookie.
Create a file middleware.ts
in the src
directory with the following code:
// File: src/middleware.ts
import { clerkMiddleware } from "astro-clerk-auth/server";
export const onRequest = clerkMiddleware();
Create the index route
Create an App.jsx
inside the src
directory with the following code:
import { SignedIn, SignedOut, UserButton } from "astro-clerk-auth/client/react";
export default function () {
return <>
<SignedOut>
<a href="/sign-in" className="rounded border px-3 py-1 max-w-max">
Sign In
</a>
</SignedOut>
<SignedIn>
<UserButton />
</SignedIn>
</>
}
The code above does the following:
- Imports the
SignedIn
andSignedOut
components that describe the state of the user whether signed in or not. - Creates conditional states, showing a
Sign In
button if the user is signed out, otherwise, renders an interactiveUserButton
component that allows a user to sign out and manage their settings.
To use this React component on the home page of your application, make the following changes in src/pages/index.astro
file:
---
// File: src/pages/index.astro
import App from "../App";
---
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<meta name="viewport" content="width=device-width" />
<meta name="generator" content={Astro.generator} />
<title>Astro</title>
</head>
<body>
<App client:load />
</body>
</html>
The changes above import the App
React component and use Astro’s client:load directive to make sure that this React component is hydrated immediately on the page.
Create a custom sign-in page
Create a sign-in.astro
file inside the src/pages
directory with the following command:
---
// File: src/pages/sign-in.astro
import { SignIn as ClerkSignIn } from "astro-clerk-auth/client/react";
---
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<meta name="viewport" content="width=device-width" />
<meta name="generator" content={Astro.generator} />
<title>Astro</title>
</head>
<body>
<ClerkSignIn client:load />
</body>
</html>
The code above does the following:
- Imports the
SignIn
component that renders all the authentication methods enabled while creating the Clerk application via the dashboard. - Use Astro’s client:load directive to make sure that this React component is hydrated immediately on the page.
Build and Test your Astro application locally
To test the application, prepare a build and run the preview server using the command below:
npm run build && node ./dist/server/entry.mjs
Conclusion
In this guide, you enabled user authentication via various methods with the help of Clerk in an Astro application. You’ve also gained some experience with using React in Astro, and understanding how it can help you build dynamic user interfaces.
If you have any questions or comments, feel free to reach out to me on Twitter.